1.Overview of Java
Thursday, August 6, 2009
Like all other computer languages, the element of java do not exists in isolation. Rather, they work together to form the language as a whole. However, this interrelation can make it difficult to describe one aspect of java without involving several others. Often a discussion of one feature implies prior knowledge of another. For this reason, this chapter presents a quick overview of several key feature of java.
Object oriented programming is at the core of the java. In fact, all java programming are object oriented. OOP is so integral to java that you must understand its basic principles before you can write even simple java programs.
Basics of Typical Java Environment
Java system generally consists of several parts; an environment, the language, the java Application Programming Interface (API) and various class libraries. The following discussion explains a typical java program development environment as shown in the fallowing diagram.
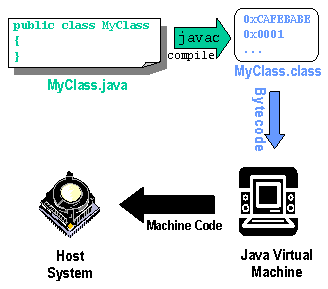
Phase 1
Phase 1 consists of editing a file. This is accomplished with an editor program. The programmer types a java program using the editor and makes corrections if necessary. When the programmer specifies that the file in the editor should save, the program is stored on a secondary storage device such as a disk. Java program file names end with the java extension. Two editors widely used on UNIX system are VI and emacs. On Windows 95 and Windows NT, simple edit programs like the DOS edit command and the windows notepad will suffice. Java's Integrated Development Environment (IDE's) such as Microsoft's Visual J++ and Symantec's visual café have built-in editors that are smoothly integrated into the programming environment.
Phase 2
In phase 2, the programmer gives the command javac to compile the program. The java compiler translates the java program into byte codes the language understood by the java interpreter.
To compile a program called welcome .java, type Javac welcome.java At the command line of your system (i.e.thru' MS-DOS prompt in windows95 and windows NT or the shell prompt in UNIX). If the program compiles correctly, the file called welcome.class is produced. This is the file containing the bytecodes that will be interpreted during the execution phase.
Java's Magic: The Byte code
The key that allows java to solve both the security and portability problems is that the output of a java compiler is not executable code. Rather, it is byte code. Byte code is a highly optimized set of instructions designed to be executed by the java runtime system, which is called the java virtual machine. That is, in its standard form, the JVM is an interpreter for the byte code. Infact, the fact that a java program is executed by the JVM helps solve the major problems associated with downloading programs associated with downloading programs over the Internet. Here is why.
Translating a java program into byte code helps makes it much easier to run a program in a wide variety of implemented for each platform. The reason is straightforward; only the JVM needs to be implemented for each platform. Once the runtime package exists for a given system, any java program can run on it. Remember that the details of the JVM will differ from platform to platform; all interpret the same java bytecode. If a java program were compiled to native code, then different versions of the same program would have to exist for each type of CPU connected to the Internet. This is of course, not a feasible solution. Thus, the interpretation of bytecode is the easiest way to create truly portable programs.
The fact a Java program is interpreted also helps to make it secure. Because the execution of every Java program is under the control of JVM, the JVM can contain the program and prevent it from generating side effects outside of the system.
When a program is interpreted, it generally runs substantially slower than it would run if compile to executable code. However, with java, the differential between the two is not so great. The use of byte code enables the Java runtime system to execute programs much faster than you might expect.
Although Java was designed for interpretation, there is technically nothing about Java that prevents on-the-fly compilation of byte code into native code. Along these lines, Sun has just completed its Just In Time (JIT) compiler for byte code, which is included in the Java 2 release. When the JIT compiler is the part of JVM it compiles byte code into executable code in the real time, on a piece-by-piece, demand basis. It is important to understand that it is not possible to compile an entire Java program into executable code all at once, because java performs various run-time checks that can be done only at runtime. Instead, the JIT compiles code, as it is needed, during execution. However the just-in-time approach still yields a significant performance boost. Even when dynamic compilation is applied to byte code, the portability and safety features still apply, because the run-time system still is in charge of the execution environment. Whether your Java program is actually interpreted in the traditional way or compiled on the fly, its functionality is the same.
Phase 3
Phase 3 is called loading. The program must first be placed in memory before it can be executed. This is done by the class loader which takes the. Class file (or files) containing the byte codes and transfers it to memory. The. Class file can be loaded from a disk on your system or a network (such as your local university or company network or even the Internet). There are two types of the programs for which the class loader loads. Class files-applications and applets. An application is a program such as a word processor program, a spreadsheet program, a drawing program, an email program etc. that is normally stored and executed from the user's local computer. An applet is a small program that is normally stored on a remote local computer that users connect to via a World Wide Web browser. Applets are loaded from a remote computer into a browser, executed in a browser and discarded when execution completed. To execute an applet again, the user must point their browser at the appropriate location on the World Wide Web and reload the program into the browser.
Applications are loaded into memory and executed using Java interpreter. When executing a Java application called Welcome. java,
the command Java Welcome Invokes the Java interpreter for the Welcome application and causes the class loader to load information used in a Welcome program.
The class loader is also executed when a Java applet is loaded into a World Wide Web browser such as Netscape's Navigator, Microsoft's Internet Explorer or Sun's Hot Java. Browsers are used to view documents on the World Wide Web, called HTML (Hyper Text Markup Language) documents. HTML is used to format a document in a manner that is easily understood by the browser application. An HTML document may refer to a Java applet. When the browser sees an applet tag in an HTML document the browser launches the Java class loader to load the applet. Applets can also be executed from the command line using the applet viewer command provided with the Java Developer's Kit (JDK)- A set of tools including the compiler (javac), interpreter (java), applet viewer and other tools used by the Java programmers. Like Netscape Navigator, Internet Explorer and Hot Java, the applet viewer requires an HTML document to invoke an applet. For example the Welcome.html file refers to the Welcome applet.
The applet viewer command is used as follows: Applet viewer Welcome.html
This causes the class loader to load the information used in the Welcome applet. The applet viewer is commonly referred to as minimum browser- it only knows how to interpret applets.
Phase 4
Before the byte code in an applet are executed by the Java interpreter built into a browser or the applet viewer, they are verified by the byte code verifier in Phase 4 (this also happens in applications that downloads byte code from a network). This ensures that the byte codes for classes that are loaded from the Internet (referred to as downloaded classes) are valid and that they do not violate Java's security because java programs arriving over the network should not be able to damage to your files and your system.
Phase 5
Finally, in Phase 5 the computer, under the control of its CPU, interpreters the program one byte code at a time, thus performing the actions specified by the program.
Programs may not work on the first try. Each of the preceding phases can fail because of the various types of errors. For example an executing program might attempt to divide by zero (an illegal operation in Java just as it is in arithmetic). This would cause the Java program to print an error messages. The programmer would return to the edit phase, make the necessary correction and proceed through the remaining phases again to determine that the corrections work properly.
A Simple Program: Printing a line of Text
We begin by considering a simple application that displays a line of text. An application is a simple program that executes using the Java interpreter. The program and its output are shown below
Steps to execute this program:
Write this program in an editor.
Compile this program by using the command javac
javac Example.java
Execute the program by using the command java
java Example
The output displayed will be: This is a simple Java program
Object oriented programming is at the core of the java. In fact, all java programming are object oriented. OOP is so integral to java that you must understand its basic principles before you can write even simple java programs.
Basics of Typical Java Environment
Java system generally consists of several parts; an environment, the language, the java Application Programming Interface (API) and various class libraries. The following discussion explains a typical java program development environment as shown in the fallowing diagram.
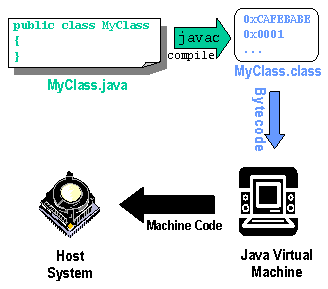
Phase 1
Phase 1 consists of editing a file. This is accomplished with an editor program. The programmer types a java program using the editor and makes corrections if necessary. When the programmer specifies that the file in the editor should save, the program is stored on a secondary storage device such as a disk. Java program file names end with the java extension. Two editors widely used on UNIX system are VI and emacs. On Windows 95 and Windows NT, simple edit programs like the DOS edit command and the windows notepad will suffice. Java's Integrated Development Environment (IDE's) such as Microsoft's Visual J++ and Symantec's visual café have built-in editors that are smoothly integrated into the programming environment.
Phase 2
In phase 2, the programmer gives the command javac to compile the program. The java compiler translates the java program into byte codes the language understood by the java interpreter.
To compile a program called welcome .java, type Javac welcome.java At the command line of your system (i.e.thru' MS-DOS prompt in windows95 and windows NT or the shell prompt in UNIX). If the program compiles correctly, the file called welcome.class is produced. This is the file containing the bytecodes that will be interpreted during the execution phase.
Java's Magic: The Byte code
The key that allows java to solve both the security and portability problems is that the output of a java compiler is not executable code. Rather, it is byte code. Byte code is a highly optimized set of instructions designed to be executed by the java runtime system, which is called the java virtual machine. That is, in its standard form, the JVM is an interpreter for the byte code. Infact, the fact that a java program is executed by the JVM helps solve the major problems associated with downloading programs associated with downloading programs over the Internet. Here is why.
Translating a java program into byte code helps makes it much easier to run a program in a wide variety of implemented for each platform. The reason is straightforward; only the JVM needs to be implemented for each platform. Once the runtime package exists for a given system, any java program can run on it. Remember that the details of the JVM will differ from platform to platform; all interpret the same java bytecode. If a java program were compiled to native code, then different versions of the same program would have to exist for each type of CPU connected to the Internet. This is of course, not a feasible solution. Thus, the interpretation of bytecode is the easiest way to create truly portable programs.
The fact a Java program is interpreted also helps to make it secure. Because the execution of every Java program is under the control of JVM, the JVM can contain the program and prevent it from generating side effects outside of the system.
When a program is interpreted, it generally runs substantially slower than it would run if compile to executable code. However, with java, the differential between the two is not so great. The use of byte code enables the Java runtime system to execute programs much faster than you might expect.
Although Java was designed for interpretation, there is technically nothing about Java that prevents on-the-fly compilation of byte code into native code. Along these lines, Sun has just completed its Just In Time (JIT) compiler for byte code, which is included in the Java 2 release. When the JIT compiler is the part of JVM it compiles byte code into executable code in the real time, on a piece-by-piece, demand basis. It is important to understand that it is not possible to compile an entire Java program into executable code all at once, because java performs various run-time checks that can be done only at runtime. Instead, the JIT compiles code, as it is needed, during execution. However the just-in-time approach still yields a significant performance boost. Even when dynamic compilation is applied to byte code, the portability and safety features still apply, because the run-time system still is in charge of the execution environment. Whether your Java program is actually interpreted in the traditional way or compiled on the fly, its functionality is the same.
Phase 3
Phase 3 is called loading. The program must first be placed in memory before it can be executed. This is done by the class loader which takes the. Class file (or files) containing the byte codes and transfers it to memory. The. Class file can be loaded from a disk on your system or a network (such as your local university or company network or even the Internet). There are two types of the programs for which the class loader loads. Class files-applications and applets. An application is a program such as a word processor program, a spreadsheet program, a drawing program, an email program etc. that is normally stored and executed from the user's local computer. An applet is a small program that is normally stored on a remote local computer that users connect to via a World Wide Web browser. Applets are loaded from a remote computer into a browser, executed in a browser and discarded when execution completed. To execute an applet again, the user must point their browser at the appropriate location on the World Wide Web and reload the program into the browser.
Applications are loaded into memory and executed using Java interpreter. When executing a Java application called Welcome. java,
the command Java Welcome Invokes the Java interpreter for the Welcome application and causes the class loader to load information used in a Welcome program.
The class loader is also executed when a Java applet is loaded into a World Wide Web browser such as Netscape's Navigator, Microsoft's Internet Explorer or Sun's Hot Java. Browsers are used to view documents on the World Wide Web, called HTML (Hyper Text Markup Language) documents. HTML is used to format a document in a manner that is easily understood by the browser application. An HTML document may refer to a Java applet. When the browser sees an applet tag in an HTML document the browser launches the Java class loader to load the applet. Applets can also be executed from the command line using the applet viewer command provided with the Java Developer's Kit (JDK)- A set of tools including the compiler (javac), interpreter (java), applet viewer and other tools used by the Java programmers. Like Netscape Navigator, Internet Explorer and Hot Java, the applet viewer requires an HTML document to invoke an applet. For example the Welcome.html file refers to the Welcome applet.
The applet viewer command is used as follows: Applet viewer Welcome.html
This causes the class loader to load the information used in the Welcome applet. The applet viewer is commonly referred to as minimum browser- it only knows how to interpret applets.
Phase 4
Before the byte code in an applet are executed by the Java interpreter built into a browser or the applet viewer, they are verified by the byte code verifier in Phase 4 (this also happens in applications that downloads byte code from a network). This ensures that the byte codes for classes that are loaded from the Internet (referred to as downloaded classes) are valid and that they do not violate Java's security because java programs arriving over the network should not be able to damage to your files and your system.
Phase 5
Finally, in Phase 5 the computer, under the control of its CPU, interpreters the program one byte code at a time, thus performing the actions specified by the program.
Programs may not work on the first try. Each of the preceding phases can fail because of the various types of errors. For example an executing program might attempt to divide by zero (an illegal operation in Java just as it is in arithmetic). This would cause the Java program to print an error messages. The programmer would return to the edit phase, make the necessary correction and proceed through the remaining phases again to determine that the corrections work properly.
A Simple Program: Printing a line of Text
We begin by considering a simple application that displays a line of text. An application is a simple program that executes using the Java interpreter. The program and its output are shown below
/* This is a simple Java program Call this file as Example. Java */
class Example
{
//your program begins with a call to the main()
public static void main(String args[])
{
System.out.println("This is a simple Java program");
}
}
Steps to execute this program:
Write this program in an editor.
Compile this program by using the command javac
javac Example.java
Execute the program by using the command java
java Example
The output displayed will be: This is a simple Java program
Labels:
Overview of Java