5.Structure of Java Program
Thursday, August 13, 2009
In this post we are going to learn structure of java program, access specifiers and modifiers and also how to create Java application in detail.
Class is blue print or an idea of an Object, from one class any number of Instances can be created and It is an encapsulation of attributes and methods.

The components of a class consists of data members (Attributes or Fields) and methods.

Creating Classes in Java:
The statements written in a Java class must end with a semicolon, ;.
Double slash, //, are comment entries. Comments are ignored by compiler.
Instance: Instance is an Object of a class which is an entity with its own attribute values and methods.
Creating an Instance
Class instance creation expressions beginning with keyword new create new objects.
For example to create an object, you need to perform the following steps:
• Declaration
• Instantiation or creation
If Employee is our class then e1 is an object of Employee

Accessing Data Members of a Class
Initially we need to assign values to data members (fields) of the object before using them. You can access the data members of a class outside the class by specifying the object name followed by the dot operator and the data member name. For example if we want to assign name for a particular employee we can use the fallowing syntax
Where e1 and e2 are the two objects or instances of a class
Adding Methods to a Class:
Performing a task in a program requires a method. Inside the method you put the mechanisms that make the method do its tasks that is the method hides the implementation details of the tasks that it performs.
A class may contain one or more methods that are designed to perform the class’s tasks. A method can perform a task and return a result. Accessing data members directly overrules the concept of encapsulation.
Advantages of using methods in a Java program:
• Re usability
• Reducing Complexity
• Data Hiding
Each message sent to an object is known as a method call and tells a method of the object to perform a task.
Each method can specify parameters that represent additional information the method requires to perform its tasks correctly. A method call supplies values called arguments for the method’s parameters.
Each parameter must specify both a type and an identifier. At the time a method is called, its arguments are assigned to its parameters, and then the method body uses the parameter variables to access the argument values.
A method can specify multiple parameters by separating each parameter from the next with a comma.
The number of arguments in the method call must match the number of parameters in the method declaration’s parameter list, also the argument types in the method call must be consistent with the types of the corresponding parameters in the method’s declaration.
The syntax to define a method:
Declaring the main() Method:
The syntax to declare the main()method:
public: method can be accessed from any object in a Java program.
static : associates the method with its class.
void: signifies that the main() method returns no value.
The main() method can be declared in any class but the name of the file and the class name in which the main() method is declared should be the same.
By convention, method names begin with a lower case first letter and all subsequent words in the name begin with a capital first letter.
The main() method accepts a single argument in the form of an array of elements of type String
When you attempt to execute a class, Java looks for the class’s main method to begin execution.
Any class that contains public static void main(String args[]) can be used to execute an application.
Typically, you cannot call a method that belongs to another class until you create an object of that class.
To call a method of an object, fallow the variable name with a dot (.) separator, the method name and a set of parenthesis containing the method’s arguments.
Installation of Java Development Kit (JDK 1.6)
You can download the most recent version of the installer from Sun’s website. Go to java.sun.com/j2se/6.0/download.jsp and click the DOWNLOAD link in the SDK column.
Just double click the installer and fallow the screen shots given below.
1.Double-click the file to begin installation. A splash screen will show for a while and then you'll be shown a licence agreement. If you want to learn Java, you must click I accept and the Next button.

2.This screen allows you to customize your installation.
The defaults shown in the upper part of the dialog box are all OK.
Change the installation directory so that all of your Java-related programs are together, as follows:
1.Click on the Change button.
2.In the resulting screen, type C:\java\jdk1.5\ in the space for the Folder name:.
3.Click OK.
You should now see the previous dialog box, shown above. Check the Install to: information to verify that it says C:\java\jdk1.5\.


3.Click Next. A progress screen will show for quite a while. It may take several minutes to fully install the software.
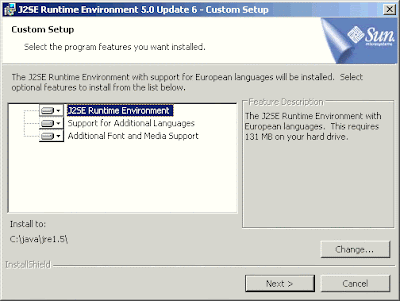
4.You will be asked to install the Java Runtime Environment. Notice that the screen is almost identical to the one in step 3. As we did there, accept the default settings except for the installation directory. Click the Change... button and change the Folder name: field to C:\java\jre1.5\. Note that this name contains jre whereas the name entered in step 3 contains jdk.
Click OK to come back to this screen. Then click Next.

5.This is your opportunity to register your Web browser with the new version of Java, enabling applets to run with the new software. Leave all of the browsers listed selected, then click Next.
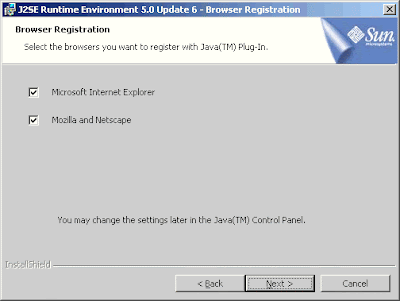
6.You will again be shown a progress screen while software is installed.
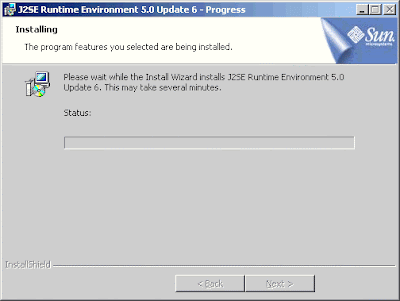
7.Eventually, you will be shown a completion screen. Click Finish.

Setting the PATH Variable
1.Open the System Properties dialog

2.Opening the Environment Variables dialog

3.Editing the PATH Variable

4.Changing the contents of the PATH Variable
You need to add C:\Program Files\Java\jdk1.5.0\bin; to the path variable at the beginning of the list.
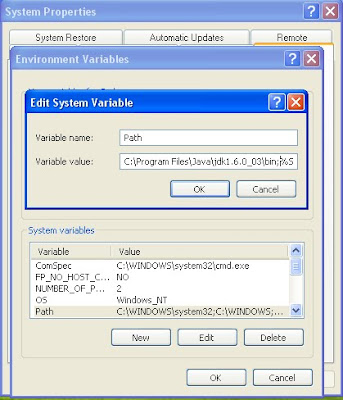
After that have a look at the fallowing examples to know more about Java Classes and Methods.
1.Text printing Java Program
2.Simple Java Program which demonstrates a class and methods in it.
In the next post, i am going to present more examples about Classes and Methods
Class is blue print or an idea of an Object, from one class any number of Instances can be created and It is an encapsulation of attributes and methods.

The components of a class consists of data members (Attributes or Fields) and methods.

Creating Classes in Java:
class
{
//Declaration of data members attributes/variables;
//Declaration of methods
Constructors();
methods();
}
The statements written in a Java class must end with a semicolon, ;.
Double slash, //, are comment entries. Comments are ignored by compiler.
Instance: Instance is an Object of a class which is an entity with its own attribute values and methods.
Creating an Instance
ClassName refVariable;
refVariable = new Constructor();
//or
ClassName refVariable = new Constructor();
Class instance creation expressions beginning with keyword new create new objects.
For example to create an object, you need to perform the following steps:
• Declaration
• Instantiation or creation
If Employee is our class then e1 is an object of Employee

Accessing Data Members of a Class
Initially we need to assign values to data members (fields) of the object before using them. You can access the data members of a class outside the class by specifying the object name followed by the dot operator and the data member name. For example if we want to assign name for a particular employee we can use the fallowing syntax
e1.employeeName="Rohith";
e2.emmployeeName="Kumar";
e1.employeeID=111;
e2.employeeID=222;
e1.employeeDesignation = “Manager”;
e2.employeeDesignation = “Salesman”;
Where e1 and e2 are the two objects or instances of a class
Adding Methods to a Class:
Performing a task in a program requires a method. Inside the method you put the mechanisms that make the method do its tasks that is the method hides the implementation details of the tasks that it performs.
A class may contain one or more methods that are designed to perform the class’s tasks. A method can perform a task and return a result. Accessing data members directly overrules the concept of encapsulation.
Advantages of using methods in a Java program:
• Re usability
• Reducing Complexity
• Data Hiding
Each message sent to an object is known as a method call and tells a method of the object to perform a task.
Each method can specify parameters that represent additional information the method requires to perform its tasks correctly. A method call supplies values called arguments for the method’s parameters.
Each parameter must specify both a type and an identifier. At the time a method is called, its arguments are assigned to its parameters, and then the method body uses the parameter variables to access the argument values.
A method can specify multiple parameters by separating each parameter from the next with a comma.
The number of arguments in the method call must match the number of parameters in the method declaration’s parameter list, also the argument types in the method call must be consistent with the types of the corresponding parameters in the method’s declaration.
The syntax to define a method:
void methodName()
{
// Method body.
}
Declaring the main() Method:
The syntax to declare the main()method:
public static void main(String args[])
{
// Code for main() method
}
public: method can be accessed from any object in a Java program.
static : associates the method with its class.
void: signifies that the main() method returns no value.
The main() method can be declared in any class but the name of the file and the class name in which the main() method is declared should be the same.
By convention, method names begin with a lower case first letter and all subsequent words in the name begin with a capital first letter.
The main() method accepts a single argument in the form of an array of elements of type String
When you attempt to execute a class, Java looks for the class’s main method to begin execution.
Any class that contains public static void main(String args[]) can be used to execute an application.
Typically, you cannot call a method that belongs to another class until you create an object of that class.
To call a method of an object, fallow the variable name with a dot (.) separator, the method name and a set of parenthesis containing the method’s arguments.
Installation of Java Development Kit (JDK 1.6)
You can download the most recent version of the installer from Sun’s website. Go to java.sun.com/j2se/6.0/download.jsp and click the DOWNLOAD link in the SDK column.
Just double click the installer and fallow the screen shots given below.
1.Double-click the file to begin installation. A splash screen will show for a while and then you'll be shown a licence agreement. If you want to learn Java, you must click I accept and the Next button.
2.This screen allows you to customize your installation.
The defaults shown in the upper part of the dialog box are all OK.
Change the installation directory so that all of your Java-related programs are together, as follows:
1.Click on the Change button.
2.In the resulting screen, type C:\java\jdk1.5\ in the space for the Folder name:.
3.Click OK.
You should now see the previous dialog box, shown above. Check the Install to: information to verify that it says C:\java\jdk1.5\.
3.Click Next. A progress screen will show for quite a while. It may take several minutes to fully install the software.
4.You will be asked to install the Java Runtime Environment. Notice that the screen is almost identical to the one in step 3. As we did there, accept the default settings except for the installation directory. Click the Change... button and change the Folder name: field to C:\java\jre1.5\. Note that this name contains jre whereas the name entered in step 3 contains jdk.
Click OK to come back to this screen. Then click Next.
5.This is your opportunity to register your Web browser with the new version of Java, enabling applets to run with the new software. Leave all of the browsers listed selected, then click Next.
6.You will again be shown a progress screen while software is installed.
7.Eventually, you will be shown a completion screen. Click Finish.
Setting the PATH Variable
1.Open the System Properties dialog

2.Opening the Environment Variables dialog

3.Editing the PATH Variable

4.Changing the contents of the PATH Variable
You need to add C:\Program Files\Java\jdk1.5.0\bin; to the path variable at the beginning of the list.
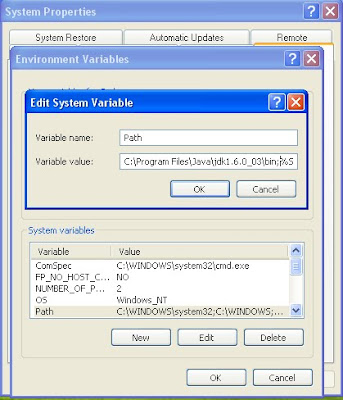
After that have a look at the fallowing examples to know more about Java Classes and Methods.
1.Text printing Java Program
// This is a simple Java Program which prints a Simple Message on to the Screen
public class Welcome
{
// main method begins execution of Java Application
public static void main(String args[])
{
System.out.println(“Welcome to JavaCircuit”);
} // end method main
} // end class Welcome
2.Simple Java Program which demonstrates a class and methods in it.
// Declaring an user defined class Employee
Class Employee
{
// Fields of the class Employee
private String employeeName;
private String employeeDesignition;
private int employeeID;
// This method is used to accept employee details
public void setEmployee(String ename,String design,int id)
{
employeeName=ename;
employeeDesignition=design;
employeeID=id;
}
// This method is used to display employee details
public void displayEmployee()
{
System.out.println(“Employee ID : “+employeeID);
System.out.println(“Employee Name : “+employeeName);
System.out.println(“Employee Designition : “+employeeDesignition);
}
}
public class EmpDemo
{
// Main method of the program
public static void main(String args[])
{
// creating object for the class Employee
Employee e=new Employee();
// Invoking the setEmployee Method to send data to the class fields
e.setEmployee(“Kiran”,”Manager”,111);
// Invoking the displayEmployee Method to display the employee details
e.displayEmployee();
}
}
In the next post, i am going to present more examples about Classes and Methods
Labels:
Structure of Java Program